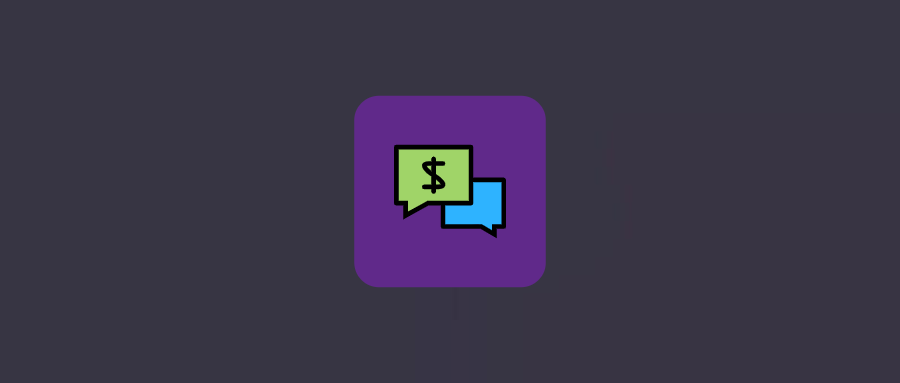
队列
队列(queue)是一种先进先出的、操作受限的线性表。必须从队尾插入新元素,队列中的元素只能从队首取出。下面是用数组实现的简单队列。
1 | // 队列 |
1 | function testQueue() { |
栈
栈是只允许在同一端进行插入和删除操作的特殊线性表。它按照先进后出的原则存储数据,先进入的数据被压入栈底,最后的数据在栈顶,需要读数据的时候从栈顶开始弹出数据。下面是使用数组实现的简单栈。
1 | class Stack { |
1 | // 测试栈 |
散列表
散列表是根据关键码值(Key value)而直接进行访问的数据结构。也就是说,它通过把关键码值映射到表中一个位置来访问记录,以加快查找的速度。这个映射函数叫做散列函数,存放记录的数组叫做散列表。
散列函数在整个过程中起着非常关键的作用,它将数据一一映射到散列表中。散列表的设计需要遵循一下规则:
- 得到的散列值是一个非负整数
- 两个相同的键,通过散列函数计算出的散列值也相同
- 两个不同的键,计算出的散列值不同
虽然我们在设计的时候要求满足以上三条要求,但是对于第三条来说,想要找到不同的 key 对应的散列值都不一样的散列函数是不可能的。即使现在非常著名的 MD5、SHA、CRC 哈希算法,也没办法避免这用哈希冲突。而且因为数组的存储空间有限,也会加大这种哈希冲突。
解决哈希冲突一般有两种方式,开放寻址法和链表法。
开放寻址法,当遇到哈希冲突的时候,在散列表中向下寻找一个空闲位置放入
链表法,在散列表每个位置存放一个链表,当出现哈希冲突的时候只需要在链表中添加一个元素即可。
下面是使用链表法实现的散列表
1 | class HashTable { |
1 | function testHashTable() { |