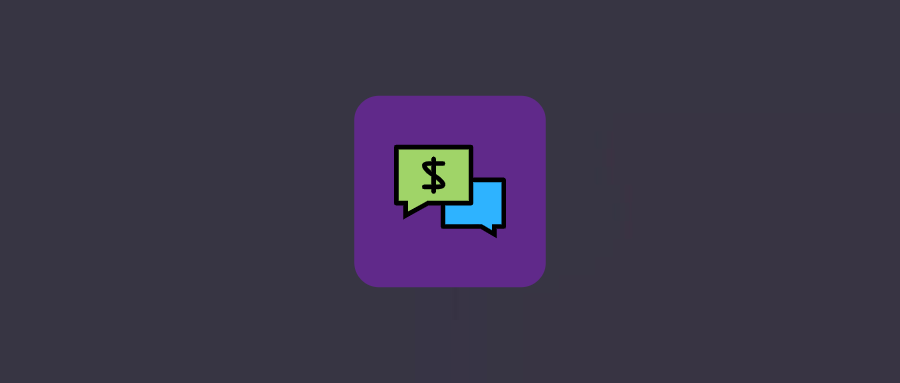
堆
堆一般来说指的是二叉堆
,在 二叉堆
中每个元素都要保证大于(小于)等于子节点的元素。当一棵二叉堆
的每个节点都符合这个条件时,它的根节点即这个二叉堆
的最大值(最小值)
使用数组来存储一棵二叉堆
,由于它是一颗完全二叉树。根据数组以及完全二叉树的特点,我们可以通过计算数组的索引在树中上下移动:从a[k]向上一层移动就令k等于k/2,向下一层移动则令k等于2k或者2k+1。利用这种数据结构我们可以实现对数级别
的插入元素
和删除最大元素
的操作。
下面是一个最大堆的实现:
1 | class Heap { |
1 | function testheap() { |